5 Ways Swap Axis
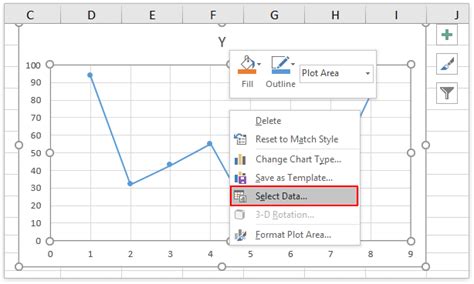
Introduction to Axis Swapping

When working with data visualizations, particularly with charts and graphs, it’s often necessary to swap the x and y axes to better represent the data or to make the visualization more intuitive. Axis swapping, or transposing, can significantly enhance the readability and understanding of the data. In this article, we’ll explore five ways to swap axes in different contexts, including Python, Excel, and more, and discuss why this technique is useful.
Understanding Axis Swapping
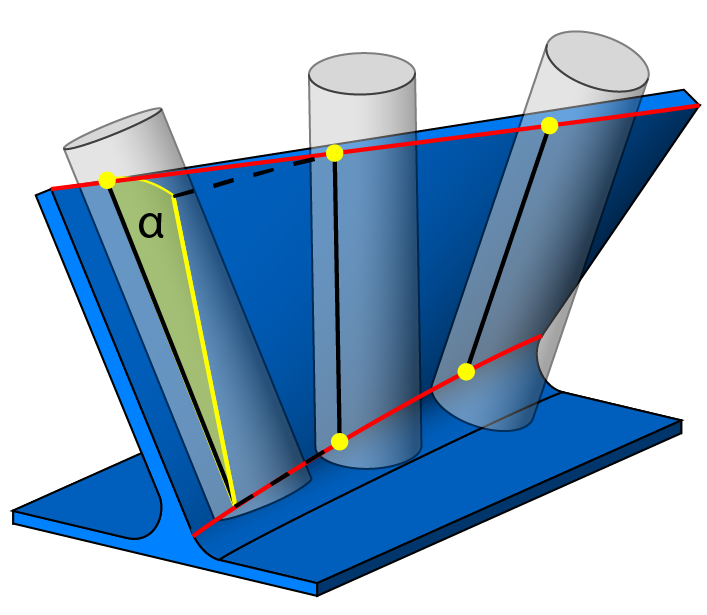
Axis swapping involves exchanging the roles of the x-axis and the y-axis in a graph. This can be particularly useful when the data’s natural orientation doesn’t match the conventional expectation. For example, if you’re plotting categories against numerical values, swapping the axes might make the graph easier to read. Before diving into the methods, it’s essential to understand that axis swapping doesn’t change the data itself but rather how it’s presented.
Method 1: Swapping Axes in Python using Matplotlib
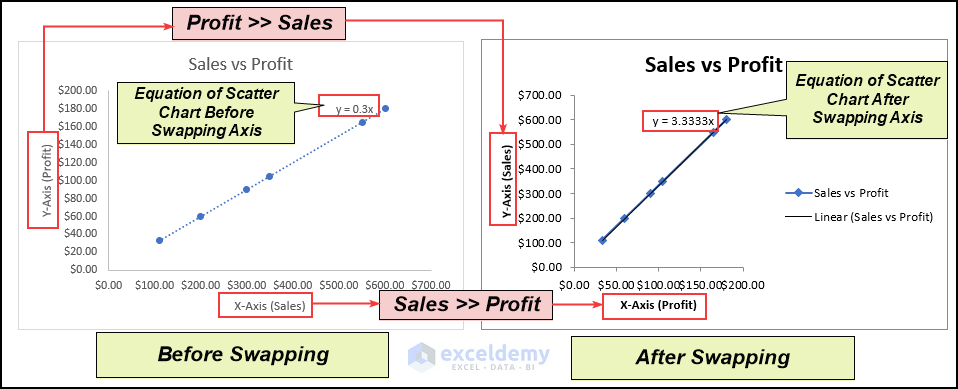
Python, with its powerful libraries like Matplotlib and Seaborn, offers straightforward ways to swap axes. When using Matplotlib, you can simply use the
transpose
function on your data before plotting, or adjust the plot function parameters to effectively swap the axes.
import matplotlib.pyplot as plt
import numpy as np
# Sample data
x = np.arange(1, 6)
y = np.array([2, 4, 6, 8, 10])
# Plotting with swapped axes
plt.plot(y, x)
plt.xlabel('Y Axis')
plt.ylabel('X Axis')
plt.show()
Method 2: Axis Swapping in Excel
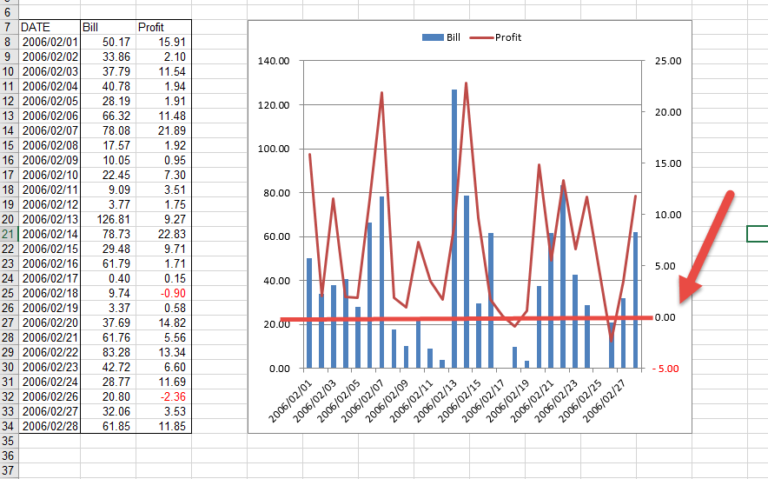
In Excel, swapping the axes of a chart can be done by switching the data ranges used for the x and y values in the chart settings. This method is useful for quick data visualization without needing to manipulate the underlying data.
- Select your chart.
- Go to the “Chart Design” tab.
- Click on “Select Data.”
- In the “Select Data Source” dialog, you can switch the series values to effectively swap the axes.
Method 3: Using Plotly for Interactive Axis Swapping

Plotly, a powerful data visualization library in Python, allows for interactive plots where axes can be swapped dynamically by the user. However, if you want to present the data with the axes initially swapped, you can adjust your data or the plot layout accordingly.
import plotly.graph_objects as go
# Sample data
x = [1, 2, 3, 4, 5]
y = [2, 4, 6, 8, 10]
# Creating a figure with swapped axes
fig = go.Figure(data=[go.Scatter(x=y, y=x)])
fig.update_layout(xaxis_title='Y Axis', yaxis_title='X Axis')
fig.show()
Method 4: Swapping Axes in Tableau
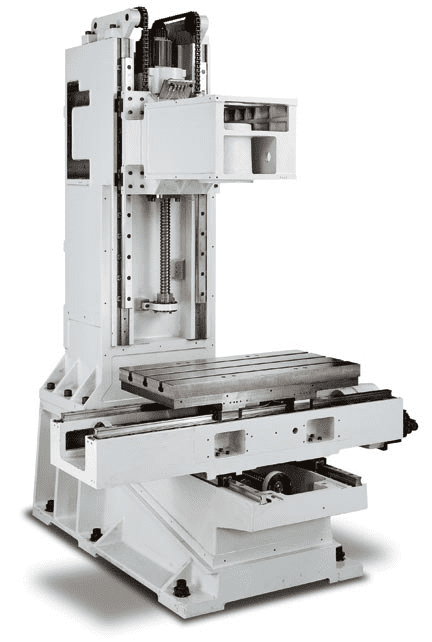
Tableau, a leading data visualization tool, makes it easy to swap axes by dragging and dropping fields in the Columns and Rows shelves. This method is highly visual and intuitive, allowing for real-time adjustments to how your data is presented.
- Drag the field currently on the Rows shelf to the Columns shelf, and vice versa.
- Tableau will automatically adjust the axes based on the new field placements.
Method 5: Axis Swapping in R using ggplot2
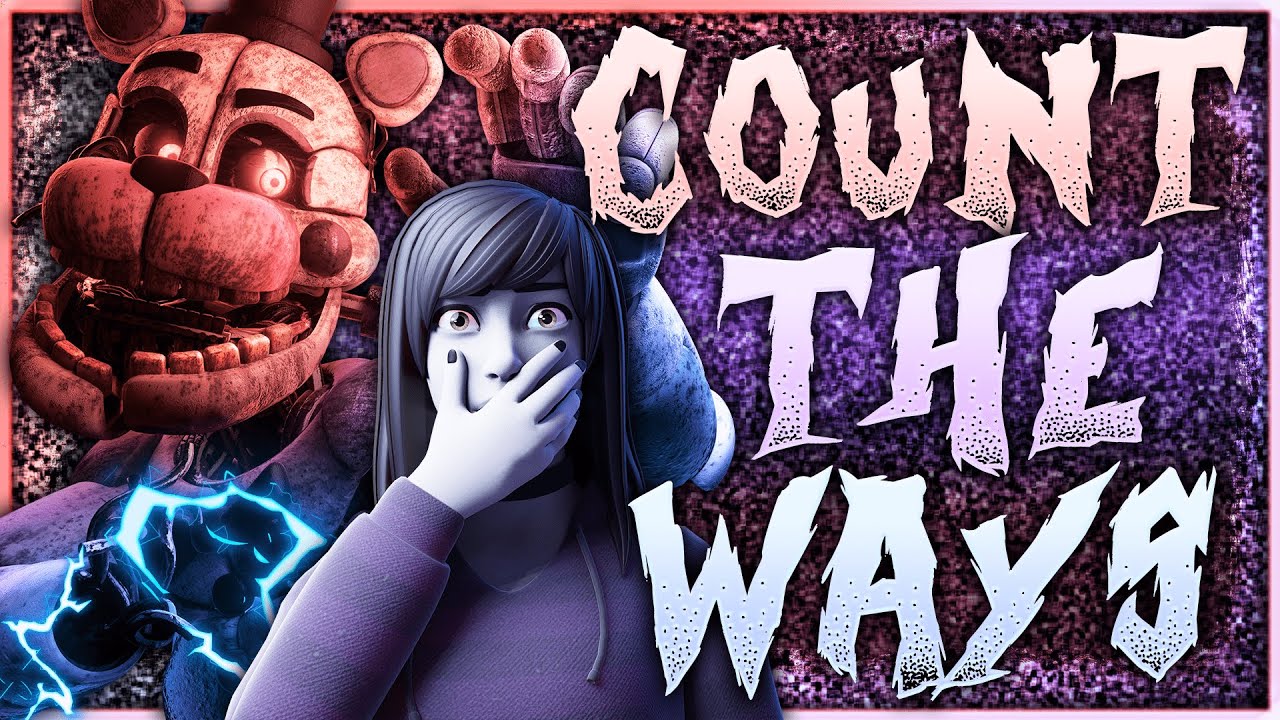
In R, the ggplot2 library provides a convenient way to create beautiful and complex data visualizations. Swapping axes in ggplot2 can be achieved by adjusting the
aes
mapping to swap x and y aesthetics.
# Install and load ggplot2 if necessary
# install.packages("ggplot2")
library(ggplot2)
# Sample data
df <- data.frame(x = c(1, 2, 3, 4, 5), y = c(2, 4, 6, 8, 10))
# Plot with swapped axes
ggplot(df, aes(x = y, y = x)) +
geom_point() +
labs(x = "Y Axis", y = "X Axis")
📝 Note: When swapping axes, ensure that the axis labels and titles are adjusted accordingly to maintain the plot's readability and accuracy.
In summary, swapping axes is a versatile technique used across various data visualization tools and programming languages to enhance the presentation and understanding of data. Whether you’re working with Python, Excel, Plotly, Tableau, or R, there are straightforward methods to achieve this. The key is understanding the data’s nature and how swapping the axes can improve the visualization’s clarity and usefulness.
What is axis swapping in data visualization?
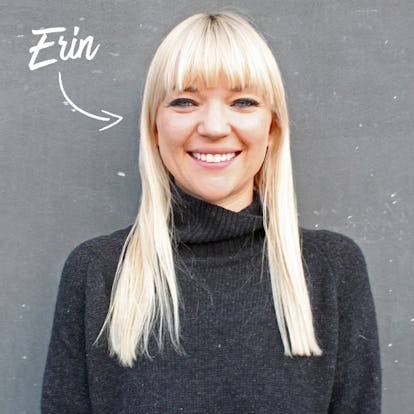
+
Axis swapping refers to the process of exchanging the roles of the x-axis and the y-axis in a graph to better represent the data or make the visualization more intuitive.
Why is axis swapping useful?

+
Axis swapping is useful because it can significantly enhance the readability and understanding of the data by presenting it in a more natural or conventional orientation.
How do I swap axes in different tools and languages?
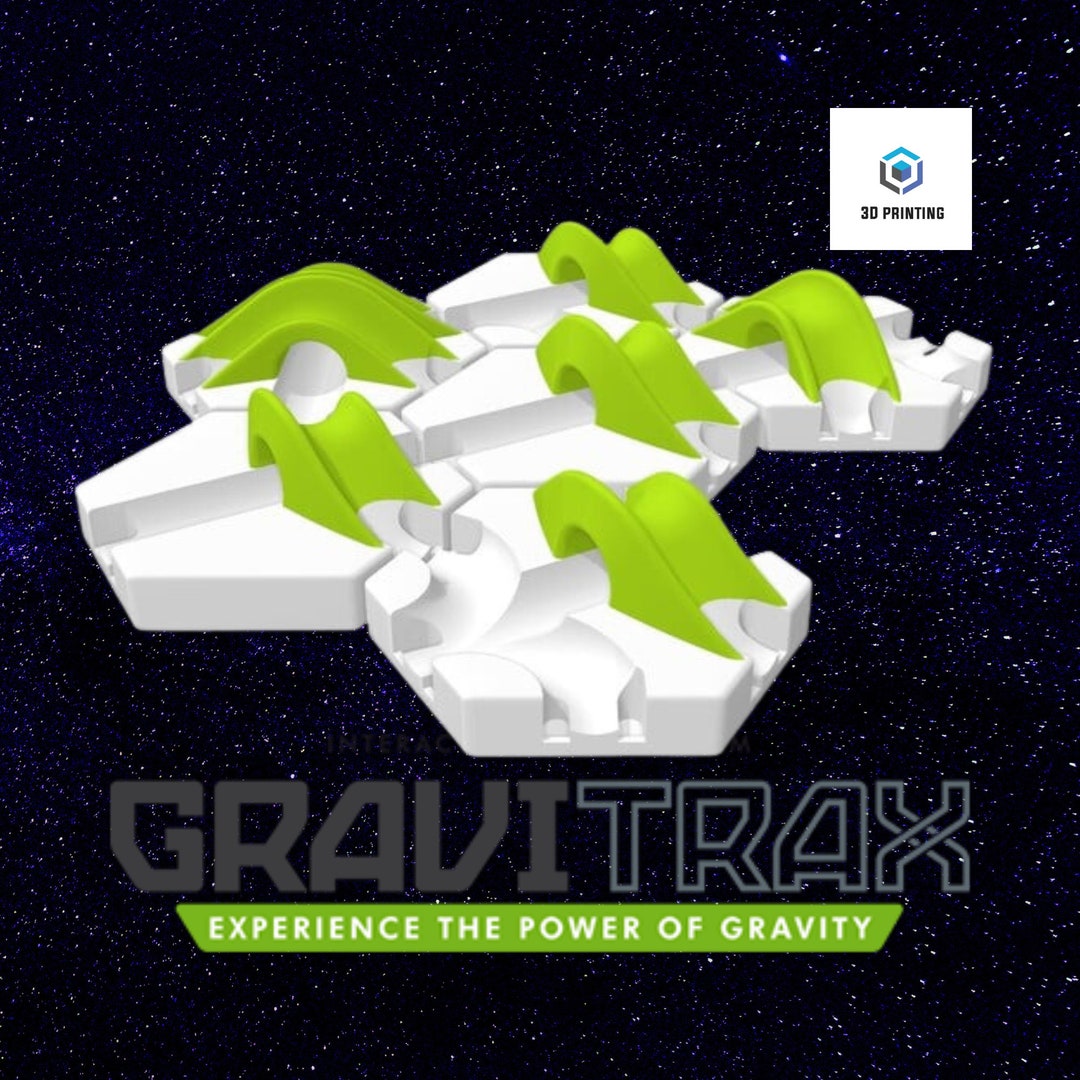
+
The method to swap axes varies by tool and language. For example, in Python with Matplotlib, you can use the plot function with the x and y values swapped. In Excel, you adjust the chart settings. In R with ggplot2, you adjust the aes mapping. Each method is tailored to the specific visualization tool or programming language being used.