5 Ways Rotate Table
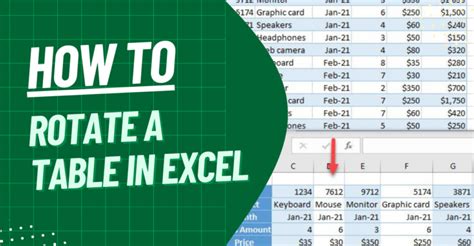
Introduction to Table Rotation
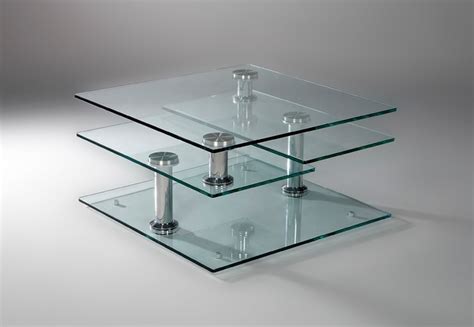
When working with tables, whether in web development, data analysis, or document preparation, being able to rotate a table can be incredibly useful. Rotation can help in better visualizing data, making it easier to read and understand, especially when dealing with tables that have more columns than rows or vice versa. In this article, we’ll explore five ways to rotate a table, covering various scenarios and tools you might encounter.
Method 1: Using CSS for Web Tables
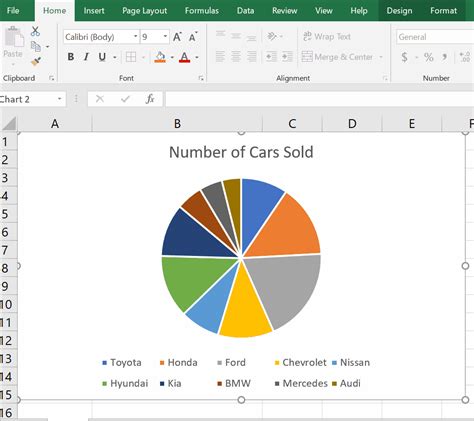
For web developers, CSS provides a straightforward way to rotate table elements. This method involves using CSS transformations to rotate the table. However, directly rotating a table element isn’t supported in standard CSS due to the table layout algorithms, but you can achieve a similar effect by rotating the content of the table cells or using the table as an image.
Here is an example of how you might rotate text within a table cell using CSS:
.rotate {
transform: rotate(90deg);
}
And then apply this class to the table cells you wish to rotate. This method doesn’t actually rotate the table structure but can give the appearance of rotated content.
Method 2: Using JavaScript for Dynamic Rotation
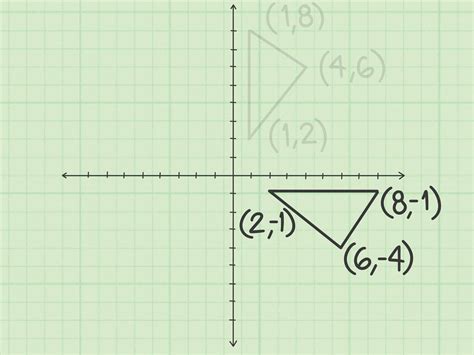
JavaScript offers more flexibility in manipulating table structures. You can write a script to transpose a table (i.e., swap rows with columns), which effectively rotates the table by 90 degrees. This involves creating a new table, iterating over the original table’s cells, and populating the new table with the cell contents in the transposed order.
For example, consider the following JavaScript snippet that creates a new table and transposes the contents of an original table:
function transposeTable(tableId) {
var table = document.getElementById(tableId);
var rows = table.rows;
var cols = rows[0].cells.length;
var newTable = document.createElement('table');
for (var i = 0; i < cols; i++) {
var newRow = newTable.insertRow();
for (var j = 0; j < rows.length; j++) {
var newCell = newRow.insertCell();
newCell.innerHTML = rows[j].cells[i].innerHTML;
}
}
// Replace the original table with the new one
table.parentNode.replaceChild(newTable, table);
}
This script can be used to dynamically rotate (transpose) tables on web pages.
Method 3: Using Microsoft Excel for Data Rotation
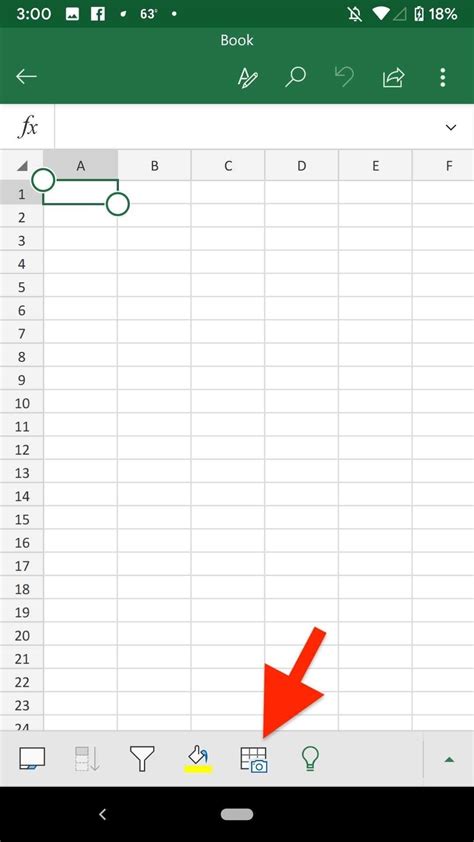
In Microsoft Excel, you can rotate a table by using the “Paste Special” feature after copying your data. This method involves: - Selecting your table and copying it. - Going to a new location in your spreadsheet where you want the rotated table to appear. - Right-clicking and selecting “Paste Special.” - Choosing “Transpose” in the Paste Special dialog box.
This will paste your data with the rows and columns swapped, effectively rotating the table.
Method 4: Using SQL for Database Table Rotation
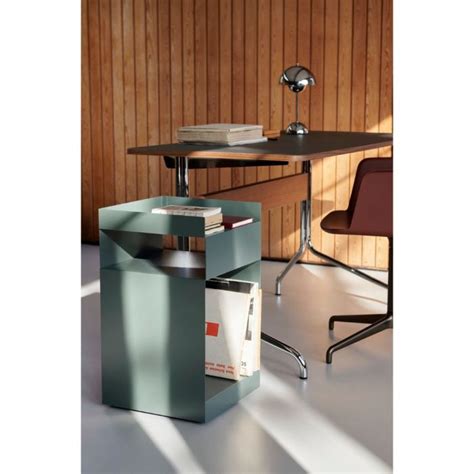
In database management systems like MySQL or PostgreSQL, you might want to rotate a table for reporting purposes or to better suit your query needs. SQL doesn’t directly support rotating tables, but you can achieve a similar effect by using pivot tables or by manually writing a query that transposes the data.
For example, if you have a simple table and you want to rotate it, you might use a query like this:
SELECT
MAX(CASE WHEN row_id = 1 THEN value END) AS col1,
MAX(CASE WHEN row_id = 2 THEN value END) AS col2,
-- Add more cases as needed
FROM your_table;
This example assumes you have a table with row identifiers and values. You’d need to adjust the query based on your table’s structure and the exact rotation you’re trying to achieve.
Method 5: Using Python for Data Frame Rotation
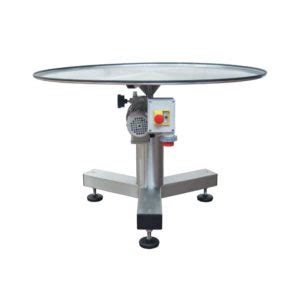
In data analysis, Python’s Pandas library provides an efficient way to manipulate data frames, which are similar to tables. You can rotate a data frame by using the
transpose()
method provided by Pandas.
Here’s a simple example:
import pandas as pd
# Create a sample data frame
data = {'Name': ['John', 'Anna', 'Peter', 'Linda'],
'Age': [28, 24, 35, 32],
'Country': ['USA', 'UK', 'Australia', 'Germany']}
df = pd.DataFrame(data)
# Transpose the data frame
df_transposed = df.transpose()
print(df_transposed)
This will output the data frame with its rows and columns swapped.
📝 Note: When working with data frames or tables in Python, always ensure your data is clean and well-structured to avoid errors during manipulation.
In conclusion, rotating a table can be achieved in various ways depending on the context and tools you’re using. Whether it’s for web development, data analysis, or database management, understanding how to transpose or rotate tables can greatly enhance your ability to work with and present data effectively.
What is the primary use of rotating a table in data analysis?
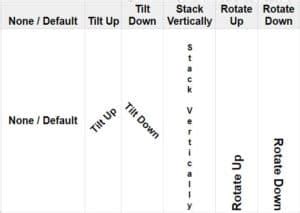
+
The primary use of rotating a table in data analysis is to better visualize and understand the data, especially when the original table structure is not intuitive for the task at hand.
Can CSS directly rotate a table element?
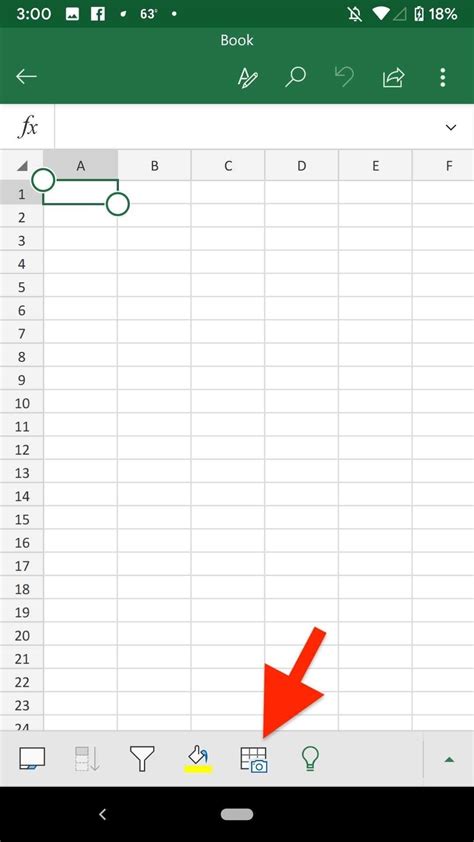
+
No, CSS does not directly support rotating table elements due to the table layout algorithms. However, you can rotate the content within table cells or use the table as an image and rotate it.
How does one rotate a table in Microsoft Excel?
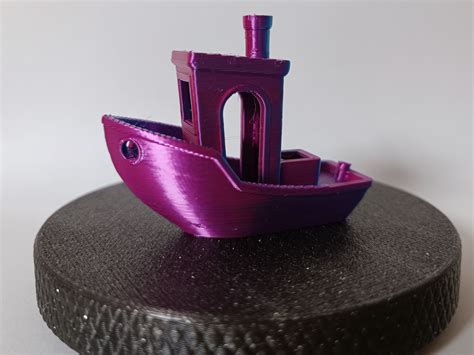
+
You can rotate a table in Microsoft Excel by copying the table, going to a new location, right-clicking, selecting “Paste Special,” and then choosing “Transpose” from the dialog box.