5 Ways Read Excel
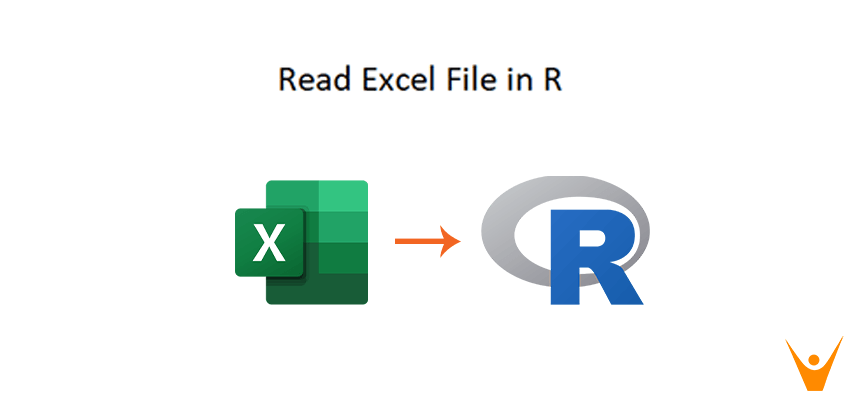
Introduction to Reading Excel Files
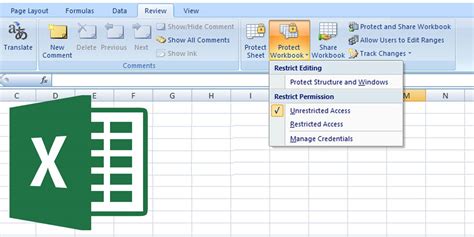
Reading Excel files is a crucial task in various applications, including data analysis, scientific computing, and business intelligence. Excel files contain a vast amount of data, and being able to read them efficiently is essential for extracting insights and making informed decisions. In this article, we will explore five ways to read Excel files, including using Python libraries, Java libraries, and other programming languages.
Method 1: Using Python Libraries
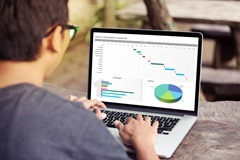
Python is a popular programming language used extensively in data analysis and scientific computing. There are several Python libraries that can be used to read Excel files, including pandas, openpyxl, and xlrd. The pandas library is one of the most widely used libraries for data analysis in Python, and it provides an efficient way to read Excel files.
import pandas as pd
# Read an Excel file
df = pd.read_excel('example.xlsx')
# Print the contents of the Excel file
print(df)
The openpyxl library is another popular library for reading and writing Excel files in Python. It provides a more low-level interface than pandas and allows for more fine-grained control over the reading and writing process.
from openpyxl import load_workbook
# Load an Excel file
wb = load_workbook('example.xlsx')
# Print the contents of the Excel file
for sheet in wb:
print(sheet.title)
Method 2: Using Java Libraries
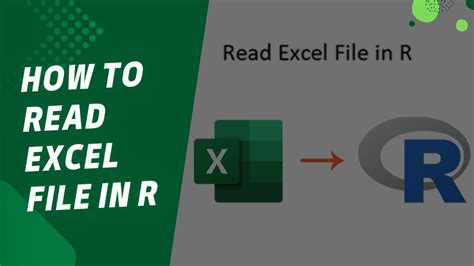
Java is another popular programming language used extensively in enterprise applications. There are several Java libraries that can be used to read Excel files, including Apache POI and JXL. The Apache POI library is one of the most widely used libraries for working with Excel files in Java, and it provides an efficient way to read and write Excel files.
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
// Read an Excel file
XSSFWorkbook wb = new XSSFWorkbook('example.xlsx');
// Print the contents of the Excel file
for (int i = 0; i < wb.getNumberOfSheets(); i++) {
System.out.println(wb.getSheetName(i));
}
The JXL library is another popular library for reading and writing Excel files in Java. It provides a more low-level interface than Apache POI and allows for more fine-grained control over the reading and writing process.
import jxl.Workbook;
import jxl.Sheet;
// Read an Excel file
Workbook wb = Workbook.getWorkbook(new File('example.xlsx'));
// Print the contents of the Excel file
for (int i = 0; i < wb.getNumberOfSheets(); i++) {
Sheet sheet = wb.getSheet(i);
System.out.println(sheet.getName());
}
Method 3: Using R Libraries
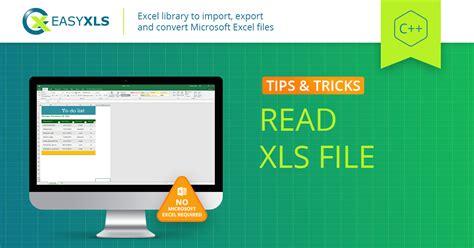
R is a popular programming language used extensively in statistical computing and data analysis. There are several R libraries that can be used to read Excel files, including readxl and xls. The readxl library is one of the most widely used libraries for reading Excel files in R, and it provides an efficient way to read Excel files.
# Install the readxl library
install.packages('readxl')
# Load the readxl library
library(readxl)
# Read an Excel file
df <- read_excel('example.xlsx')
# Print the contents of the Excel file
print(df)
The xls library is another popular library for reading and writing Excel files in R. It provides a more low-level interface than readxl and allows for more fine-grained control over the reading and writing process.
# Install the xls library
install.packages('xls')
# Load the xls library
library(xls)
# Read an Excel file
df <- read.xls('example.xlsx')
# Print the contents of the Excel file
print(df)
Method 4: Using Node.js Libraries
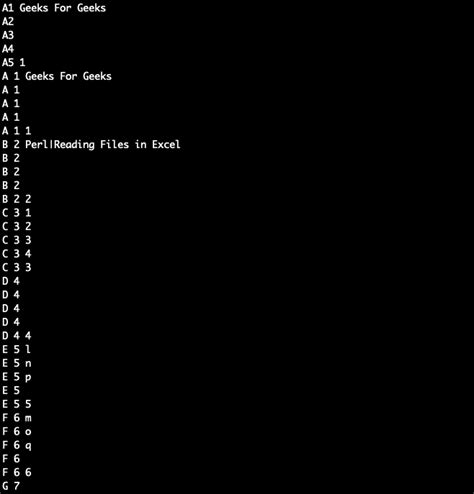
Node.js is a popular programming language used extensively in web development and server-side applications. There are several Node.js libraries that can be used to read Excel files, including exceljs and xlsx. The exceljs library is one of the most widely used libraries for reading and writing Excel files in Node.js, and it provides an efficient way to read Excel files.
// Install the exceljs library
npm install exceljs
// Load the exceljs library
const ExcelJS = require('exceljs');
// Read an Excel file
const wb = new ExcelJS.Workbook();
wb.xlsx.readFile('example.xlsx').then(() => {
// Print the contents of the Excel file
console.log(wb.sheetNames);
});
The xlsx library is another popular library for reading and writing Excel files in Node.js. It provides a more low-level interface than exceljs and allows for more fine-grained control over the reading and writing process.
// Install the xlsx library
npm install xlsx
// Load the xlsx library
const XLSX = require('xlsx');
// Read an Excel file
const wb = XLSX.readFile('example.xlsx');
// Print the contents of the Excel file
console.log(wb.SheetNames);
Method 5: Using MATLAB
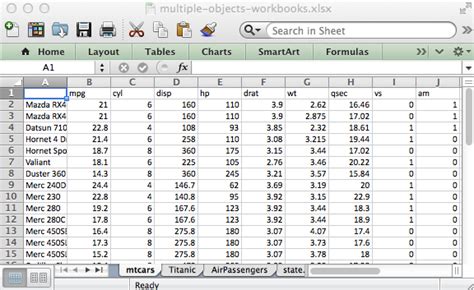
MATLAB is a popular programming language used extensively in numerical computing and data analysis. MATLAB provides a built-in function called readtable that can be used to read Excel files.
% Read an Excel file
tbl = readtable('example.xlsx');
% Print the contents of the Excel file
disp(tbl);
In addition to the readtable function, MATLAB also provides a built-in function called xlsread that can be used to read Excel files.
% Read an Excel file
[num, txt, raw] = xlsread('example.xlsx');
% Print the contents of the Excel file
disp(num);
disp(txt);
disp(raw);
📝 Note: The above methods assume that the Excel file is in the same directory as the script. If the Excel file is located in a different directory, the file path needs to be specified.
As we have seen, there are several ways to read Excel files using different programming languages and libraries. Each method has its own strengths and weaknesses, and the choice of method depends on the specific requirements of the application. By using the right library or function, developers can efficiently read Excel files and extract the data they need.
The key points to take away from this article are: * There are several programming languages and libraries that can be used to read Excel files, including Python, Java, R, Node.js, and MATLAB. * Each library or function has its own strengths and weaknesses, and the choice of method depends on the specific requirements of the application. * The pandas library in Python and the Apache POI library in Java are two of the most widely used libraries for reading Excel files. * The readxl library in R and the exceljs library in Node.js are also popular libraries for reading Excel files. * MATLAB provides built-in functions like readtable and xlsread to read Excel files.
In summary, reading Excel files is an essential task in various applications, and there are several programming languages and libraries that can be used to accomplish this task. By choosing the right library or function, developers can efficiently read Excel files and extract the data they need.
What is the most widely used library for reading Excel files in Python?
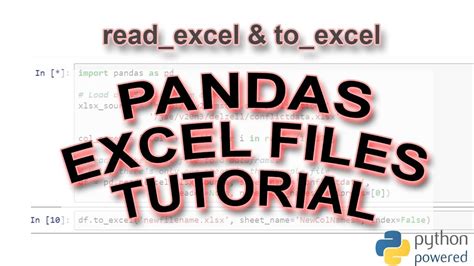
+
The pandas library is the most widely used library for reading Excel files in Python.
What is the most widely used library for reading Excel files in Java?
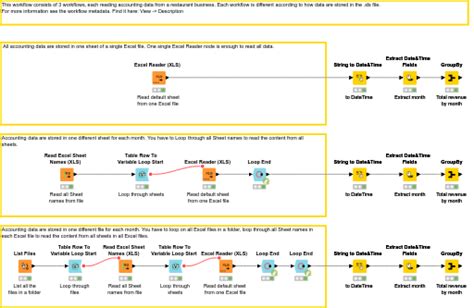
+
The Apache POI library is the most widely used library for reading Excel files in Java.
Can MATLAB be used to read Excel files?
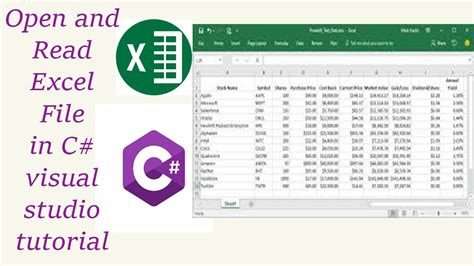
+
Yes, MATLAB can be used to read Excel files using built-in functions like readtable and xlsread.