5 Ways Convert Date
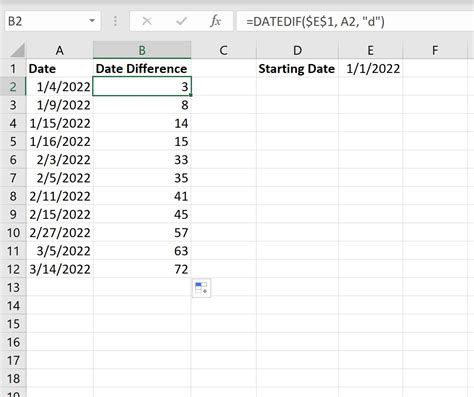
Introduction to Date Conversion
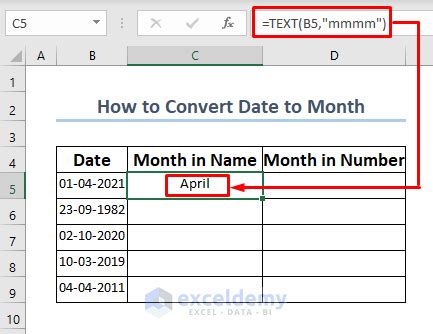
Converting dates from one format to another is a common task in various applications, including web development, data analysis, and software engineering. Dates can be represented in different formats, such as MM/DD/YYYY, DD/MM/YYYY, or YYYY-MM-DD, and converting them correctly is essential to ensure data consistency and accuracy. In this article, we will explore 5 ways to convert dates in different programming languages and tools.
Method 1: Using JavaScript
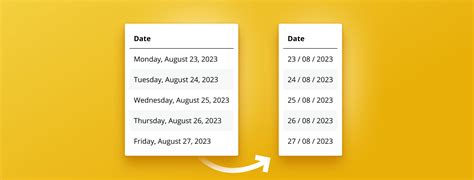
JavaScript provides several methods to convert dates, including the use of the Date object and its various methods. One way to convert a date is to use the toLocaleDateString method, which returns a string representing the date in a specific format. For example:
let date = new Date('2022-07-25');
let formattedDate = date.toLocaleDateString('en-US', { year: 'numeric', month: '2-digit', day: '2-digit' });
console.log(formattedDate); // Output: 07/25/2022
This method is useful for converting dates to a specific format, such as MM/DD/YYYY.
Method 2: Using Python
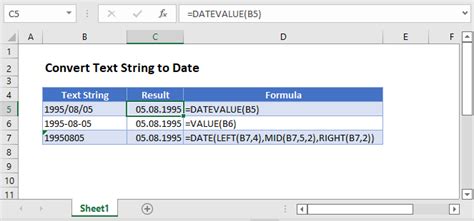
Python provides the datetime module, which includes classes for manipulating dates and times. The strftime method can be used to convert a date to a specific format. For example:
from datetime import datetime
date = datetime.strptime('2022-07-25', '%Y-%m-%d')
formatted_date = date.strftime('%m/%d/%Y')
print(formatted_date) # Output: 07/25/2022
This method is useful for converting dates to a specific format, such as MM/DD/YYYY.
Method 3: Using Excel
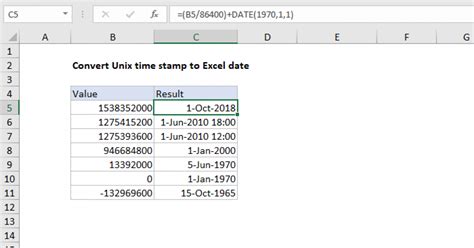
Excel provides several functions to convert dates, including the TEXT function. This function can be used to convert a date to a specific format, such as MM/DD/YYYY. For example:
=TEXT(A1,"mm/dd/yyyy")
Assuming the date is in cell A1, this formula will return the date in the format MM/DD/YYYY.
Method 4: Using SQL
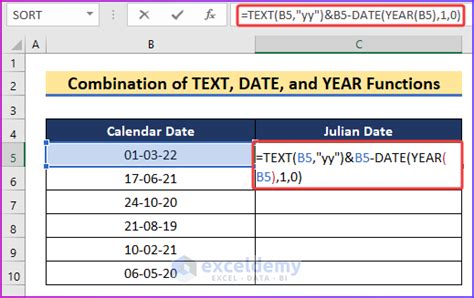
SQL provides several functions to convert dates, including the FORMAT function. This function can be used to convert a date to a specific format, such as MM/DD/YYYY. For example:
SELECT FORMAT(date, 'MM/dd/yyyy') AS formatted_date
FROM table_name;
This method is useful for converting dates to a specific format, such as MM/DD/YYYY.
Method 5: Using Online Tools
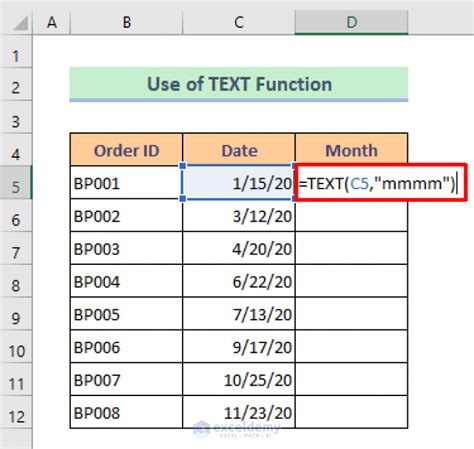
There are several online tools available that can be used to convert dates, such as online date converters or calendar tools. These tools can be useful for quickly converting dates without having to write code or use a specific software application.
📝 Note: When converting dates, it's essential to consider the format of the input date and the desired output format to ensure accurate conversion.
In conclusion, converting dates from one format to another is a common task that can be accomplished using various programming languages and tools. By using the methods outlined in this article, you can easily convert dates to the desired format, ensuring data consistency and accuracy.
What is the most common date format used in the United States?
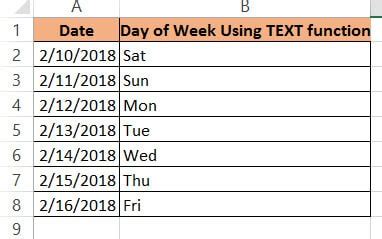
+
The most common date format used in the United States is MM/DD/YYYY.
How do I convert a date from YYYY-MM-DD to MM/DD/YYYY using JavaScript?
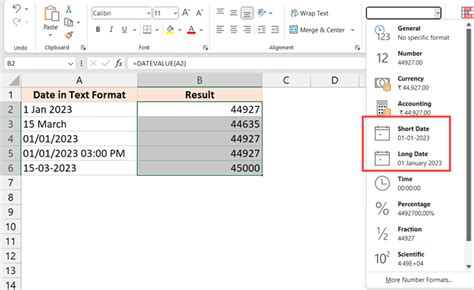
+
You can use the toLocaleDateString method to convert a date from YYYY-MM-DD to MM/DD/YYYY. For example: let date = new Date(‘2022-07-25’); let formattedDate = date.toLocaleDateString(‘en-US’, { year: ‘numeric’, month: ‘2-digit’, day: ‘2-digit’ });
What is the purpose of the datetime module in Python?
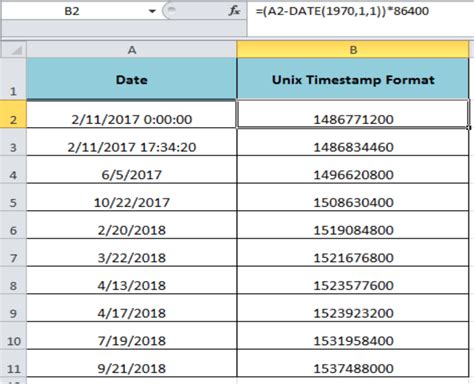
+
The datetime module in Python provides classes for manipulating dates and times. It can be used to convert dates to specific formats, perform date arithmetic, and more.