5 Ways Remove Space
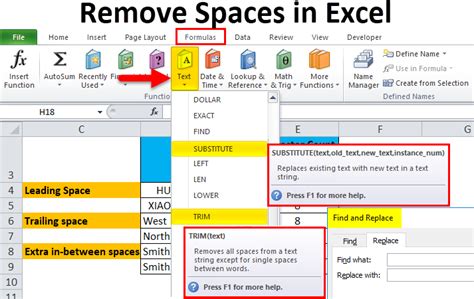
Introduction to Removing Spaces
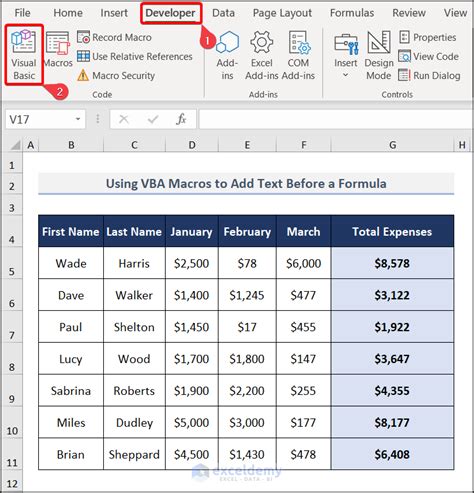
Removing unwanted spaces from text or data is a common task in various fields, including data analysis, programming, and document editing. Unnecessary spaces can make text harder to read, increase file sizes, and complicate data processing. In this article, we will explore five ways to remove spaces, focusing on methods applicable to text editing, programming, and data manipulation.
Method 1: Using Text Editors
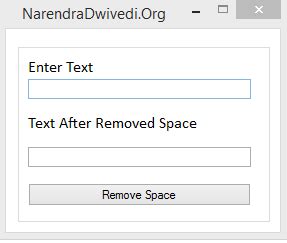
Most text editors, including Notepad, TextEdit, and advanced editors like Sublime Text or Visual Studio Code, provide functionalities to remove spaces. Here are the general steps: - Open your document in the text editor. - Use the Find and Replace feature, usually accessible via Ctrl + H (Windows) or Cmd + H (Mac). - In the find box, type a space to search for spaces. - Leave the replace box empty if you want to remove all spaces. - Click Replace All to remove all spaces.
Method 2: Using Microsoft Word
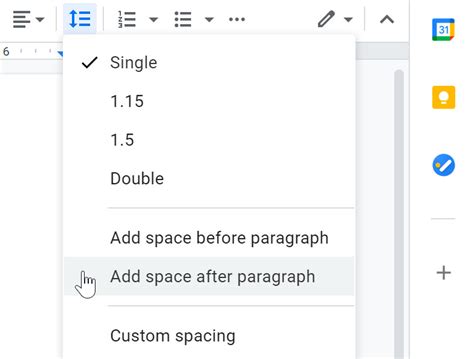
Microsoft Word offers several ways to remove spaces, including using its built-in Find and Replace tool or adjusting paragraph settings. - To remove extra spaces between sentences or paragraphs: - Select the text. - Right-click and choose Paragraph. - Adjust the Spacing options as needed. - For removing all spaces: - Use the Find and Replace feature (Ctrl + H). - Type ^w in the find box to search for words (this method removes spaces between words but keeps words intact). - Leave the replace box empty and click Replace All.
Method 3: Using Programming Languages
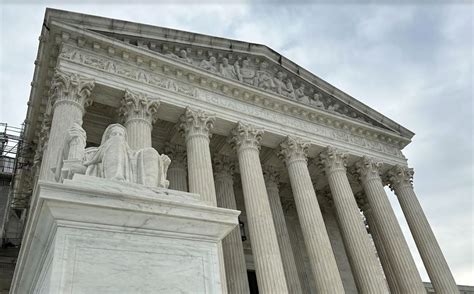
In programming, spaces can be removed using string manipulation functions. For example, in Python:
text = "Hello World"
text_without_spaces = text.replace(" ", "")
print(text_without_spaces)
This code outputs: HelloWorld.
Method 4: Using Online Tools
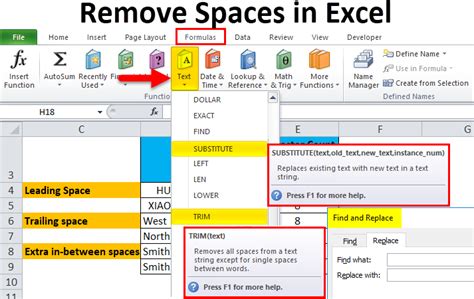
Several online tools and websites offer space removal services. These tools are handy for quick edits without the need to open a text editor or programming environment. - Visit a website that offers a “remove spaces” tool. - Paste your text into the input box. - Click the Remove Spaces button. - Copy the output.
Method 5: Using Regular Expressions
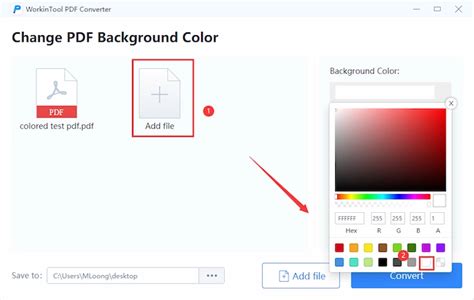
Regular expressions (regex) provide a powerful way to search and manipulate patterns in text, including removing spaces. - In JavaScript, for example, you can use:
let text = "Remove spaces from this text";
text = text.replace(/\s+/g, '');
console.log(text);
This will output: Removespacesfromthistext.
📝 Note: When using regex or any programming method, be cautious with the input data to avoid unexpected results.
To summarize, removing spaces can be achieved through various methods, each suitable for different contexts and needs. Whether you’re working with text editors, programming languages, online tools, or Microsoft Word, there’s a straightforward way to eliminate unwanted spaces and make your text more compact and readable.
What is the easiest way to remove all spaces from a text file?
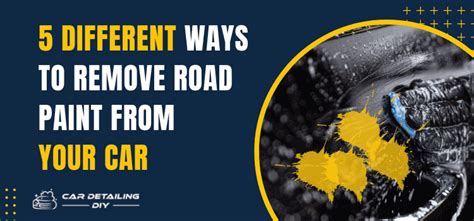
+
The easiest way often involves using a text editor’s Find and Replace feature, where you find all spaces and replace them with nothing, effectively removing them.
Can I remove spaces from a document using Microsoft Word?
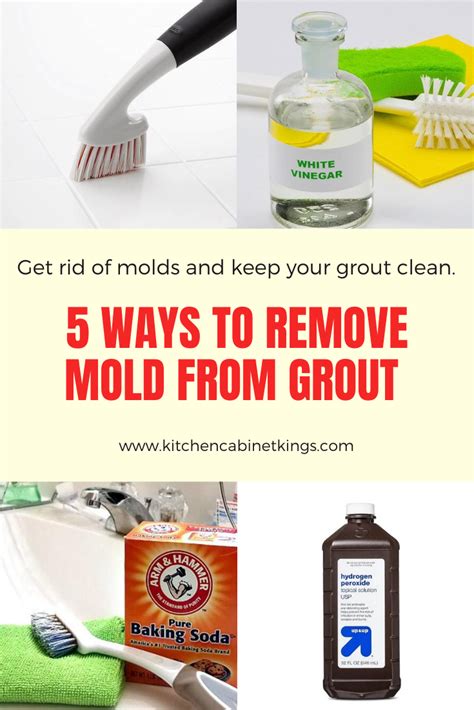
+
Yes, Microsoft Word offers several methods to remove or adjust spaces, including using the Find and Replace tool or adjusting paragraph settings.
How do I remove spaces in a string using Python?
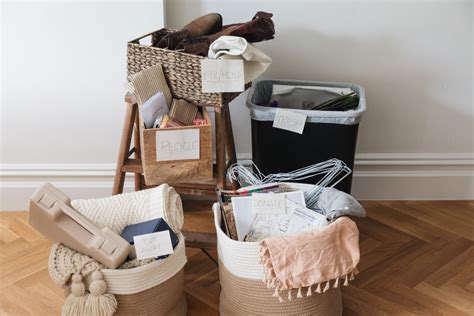
+
You can use the replace() method in Python, like so: my_string.replace(” “, “”), to remove all spaces from a string.