5 Ways Hide Div 0

Introduction to Hiding Div Elements
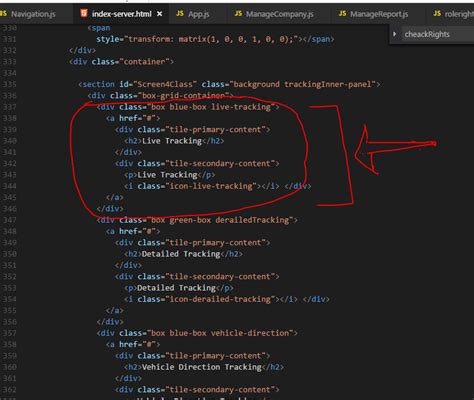
When working with web development, particularly with HTML and CSS, there are several scenarios where you might want to hide a div element. This could be for reasons such as displaying content conditionally based on user interactions, managing layout space, or enhancing user experience by controlling the visibility of certain elements. In this post, we will explore five common methods to hide a div element, each serving different purposes and use cases.
Method 1: Using the display
Property
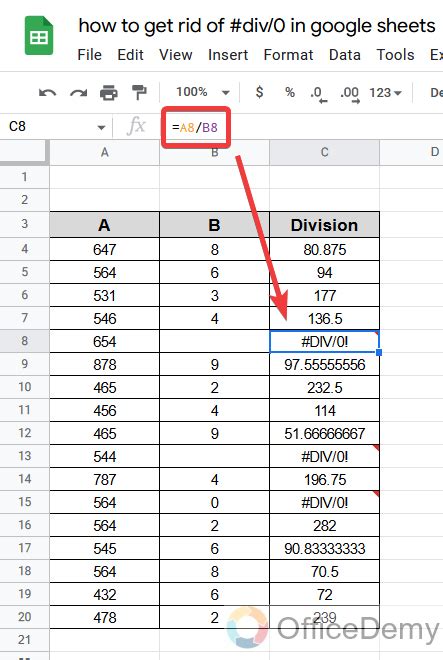
One of the most straightforward ways to hide a div is by using the
display
property in CSS. By setting display: none;
, you can completely remove the element from the layout, meaning it will not occupy any space on the page. This method is useful when you want to completely remove an element from the page flow.
#myDiv {
display: none;
}
📝 Note: Using `display: none;` will completely remove the element from the DOM in terms of layout, meaning it won't take up space, and it won't be accessible for screen readers or other assistive technologies.
Method 2: Using the visibility
Property
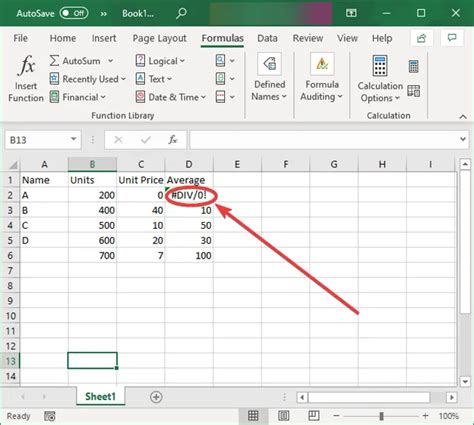
Another method to hide a div is by using the
visibility
property. Setting visibility: hidden;
will make the element invisible, but unlike display: none;
, it will still occupy space in the layout. This can be useful in scenarios where you want to hide an element but keep its space reserved.
#myDiv {
visibility: hidden;
}
Method 3: Using the opacity
Property
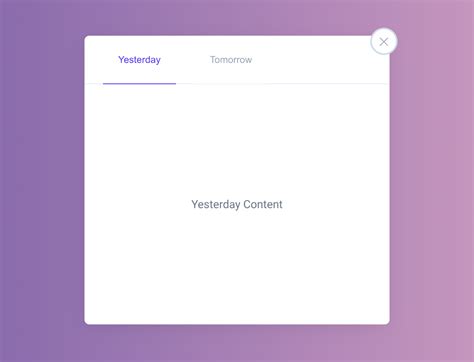
You can also hide a div by adjusting its opacity. Setting
opacity: 0;
will make the element completely transparent, effectively hiding it from view. However, similar to visibility: hidden;
, the element will still occupy space in the layout and will still be interactive.
#myDiv {
opacity: 0;
}
Method 4: Using height
and width
Properties
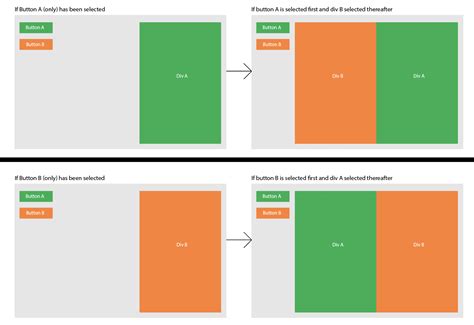
Another approach to hiding a div involves setting both its
height
and width
to 0
, and also setting overflow
to hidden
. This method effectively collapses the element, removing it from view, though it may still be present in the DOM and could potentially be interacted with via screen readers or direct DOM manipulation.
#myDiv {
height: 0;
width: 0;
overflow: hidden;
}
Method 5: Using position
and left
Properties
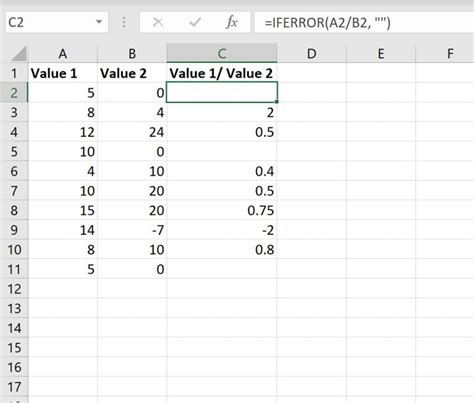
The final method involves moving the div off-screen. By setting
position: absolute;
and then moving it far to the left (e.g., left: -9999px;
), you can effectively hide the div from the user’s view. This method is useful for accessibility purposes, such as hiding content that should only be read by screen readers.
#myDiv {
position: absolute;
left: -9999px;
}
In summary, each method for hiding a div element has its own set of use cases and implications for layout, accessibility, and user experience. Choosing the right method depends on your specific needs, such as whether you want the element to still occupy space, be accessible to screen readers, or be completely removed from the layout.
What is the difference between display:none and visibility:hidden?
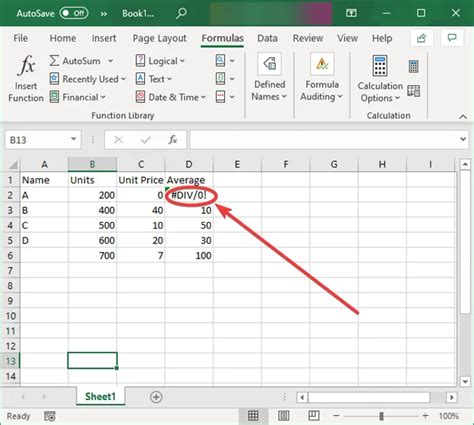
+
The key difference is that display:none
removes the element from the layout, while visibility:hidden
hides the element but still occupies space in the layout.
How does using opacity affect the element’s interactivity?
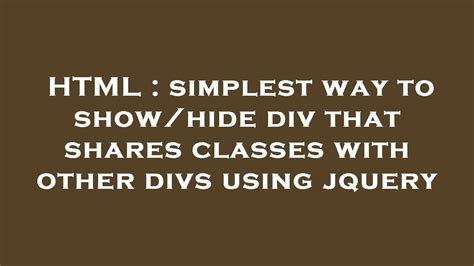
+
Setting opacity: 0;
makes the element invisible but does not prevent it from being interactive. Users can still click on or interact with the invisible element.
Why would you use position:absolute and left:-9999px to hide an element?
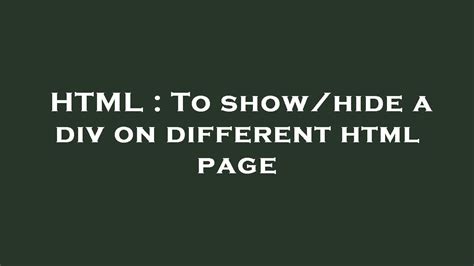
+
This method is primarily used for accessibility. It moves the element off-screen, making it invisible to users but still accessible to screen readers, allowing for screen reader users to access the content.